In general the process of menu creation consists of the following stages:
- defining HTML elements used in menu template;
- menu template creating;
- including function for menu call in site template (in template prolog or epilog);
- creating menu description file (file with menu items).
Menu structure
Any menu of a site is built based on the two components:
- array of data provided in the administrative section (the product ships with the demo menu);
- menu design template.
Array of data defines the menu composition, text and links of all the menu items. A menu template is the PHP code that describes the menu design. Menu template processes the data array and generates the HTML code.
Array of data
Files with menu items are located in those sections (directories) where they are to be used for the menu display. This files have the following names: .<menu_type>.menu.php
.
For example, for storing data of menu with type "left" will be used file .left.menu.php
, and for data of menu with type "top" will be used file .top.menu.php
.
If a given section does not contain a menu description file, the system will search a section of upper level for such files.
E.g. usually the main site menu is considered to be displayed in all site sections (in the product demonstrational version it is menu with type "top"). That is why this menu description file is stored only in the site root directory.
Also usually it is supposed that every site section should have its own complementary menu. For this purpose description file for complementary menu is created in every site section:
The Bitrix Site Manager allows using dynamic menu. The data array for such menu is generated automatically accordingly to data get wit use of the special program script. This scrip must be stored in file with the mane ..menu_ext.php
in folder of the corresponding site section.
In this case the "Mobile phones" and "Accessories" information block section names are used as menu item. A program script collecting data for dynamic menu is stored in the file .left.menu_ext.php
in folders of the corresponding site sections.
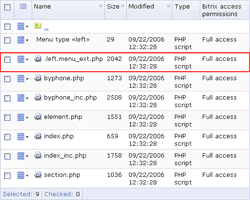
Menu design template
Template developing
The first thing you need to do to customize the template is to choose the repeating parts of a menu.
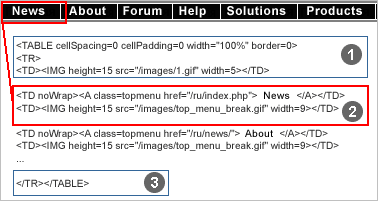
For instance, the horizontal menu is comprised of table cells, while the vertical menu consists of rows.
Menu template creating
All menu templates share the common structure:
- menu template header part;
- description of substitutions for different processing conditions;
- menu template body;
- menu template footer part.
Let us consider how a template can be constructed by the example of left menu:
<?
//--- The following variables can be used when creating a menu template:
//--- $TEXT – menu item text;
//--- $LINK – link URL of the menu item;
//--- $ITEM_TYPE – menu item type. Can have the following values:
//--- "D" – directory, "P" - page, "U" – parametrized page.
//--- $ITEM_INDEX – menu item index.
//--- $SELECTED – set to true if the menu item is selected.
//--- $PERMISSION – access permissions for the current page.
//--- Can have the following values: "D" – access denied, "R" – read,
//--- "W" – write, "X" – write and permission modification.
//--- $PARAMS["<parameter>"] – array of parameter values
//--- set in the extended editing mode.
//------------- Menu Header -------------------------------------
//--- Upper constant part of the table.
$sMenuProlog="<table width='100%' border='0' cellspacing='0' cellpadding='0' background=''>n";
//--- Description of substitutions for different processing conditions
//--- Template elements are altered according to the display conditions.
//--- If any menu item is set to display as the separator,
//--- a corresponding parameter value is checked:
//--- $PARAMS["SEPARATOR"]=="Y".
//--- Parameter "SEPARATOR" can be assigned
//--- when editing the menu in the extended mode.
//--- Other parameters are optional and can be set as desired.
//--- Please note that a parameter named "SEPARATOR"
//--- is also checked when building a site map.
//--- Certain alterations are made to template elements
//--- according to conditions.
if ($PARAMS["SEPARATOR"]=="Y")
{
$clrbg = " class=’menuborder’";
$clrtext = "leftmenub";
}
else
{
$clrbg = "";
$clrtext = "leftmenu";
}
if($SELECTED)
{
$clrtext = "leftmenuact";
}
else
{
$clrtext = "leftmenu";
}
if ($ITEM_TYPE=="D")
{
$clrimg = " <img src=’/images/arrows_r.gif’ width=’11’ height=’9’ border=’0’>";
}
else
{
$clrimg = "";
}
//------------- Menu template body -------------------------------------
//--- This section describes a single element of the common structure,
//--- i.e. a single menu item. All menu items are generated in a loop.
//--- Template elements that can vary depending on conditions,
//--- are represented by variables, e.g. $clrbg, $clrimg, $clrtext.
//--- The mandatory parameters (menu item text and link)
//--- are described by the variables $TEXT and $LINK, respectively.
//--- Vriable $PARAMS["ALT"] contains the value of the "ALT" attribute
//--- specified in the extended editing mode.
//--- User permissions are checked before generating a menu item.
if ($PERMISSION>"D")
{
$sMenuBody =
"<tr>n".
"<td width='0%' ". $clrbg ." nowrap valign="top" ><img src='/images/1.gif' width='2' height='8'><img src='/images/1.gif' width='30' height='15'></td>n".
"<td width=’100%’ ". $clrbg ."><a href='".$LINK."' class='".$clrtext."' title='".$PARAMS["ALT"]."'>".$TEXT."". $clrimg ."</a></td>n".
"<td width='0%' ". $clrbg ."><img src='/images/1.gif' width='5' height='1'></td>n".
"</tr>n";
}
else
{
$sMenuBody =
"<tr>n".
"<td width='0%' ". $clrbg ." nowrap valign="top" ><img src='/images/1.gif' width='2' height='8'><img src=’/images/1.gif' width='30' height='15'></td>n".
"<td width='100%' ". $clrbg .">".$TEXT."". $clrimg ."</td>n".
"<td width=’0%’ ". $clrbg ."><img src='/images/1.gif' width='5' height='1'></td>n".
"</tr>n";
}
//------------- Menu template footer -------------------------------------
//--- Table closing tag. Bottom constant part.
$sMenuEpilog="</table>";
?>
The repeating portion of a menu defined on the previous step is placed in the template body.
Developing a menu template you may need to create additional stylesheets (CSS). For example, for text menu additional CSS can define menu items color, color of the current menu item, and etc.
Also some menu items (for example, section titles) can be displayed in a special way. More over there can be used graphical or text element for indication active or unavailable menu items, or designation that the menu item refers to subsections or document of the current section.
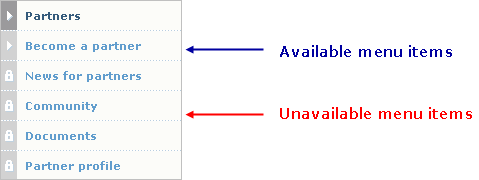
Templates for top and left menu, included in the system distributive, are stored in the directory /bitrix/templates/<site_id>/
in files top.menu_template.php
and left.menu_template.php
correspondingly.
Menu show
Resulting menus can be incorporated in the design template by calling the following functions:
<?
//--- Upper menu inclusion code
echo $APPLICATION->GetMenuHtml("top");
?>
…
<?
//--- Left menu inclusion code
echo $APPLICATION->GetMenuHtml("left");
?>
This function is placed in the template areas assigned for menu show.
Menu is built in the following way. A common design template calls the function that generates the code responsible for the menu display. When a page is being loaded, this function checks for presence of a menu description file in the given section; calls the template of the corresponding type to build the menu; generates the HTML code of the menu