Example for creating an agent
In case when you need to dynamically add specific agents, use the agent API. Be advised, that it's easier to add one or two agents manually.
Go to the page Settings > Product Settings > Agents and click Add an Agent button on the context panel:
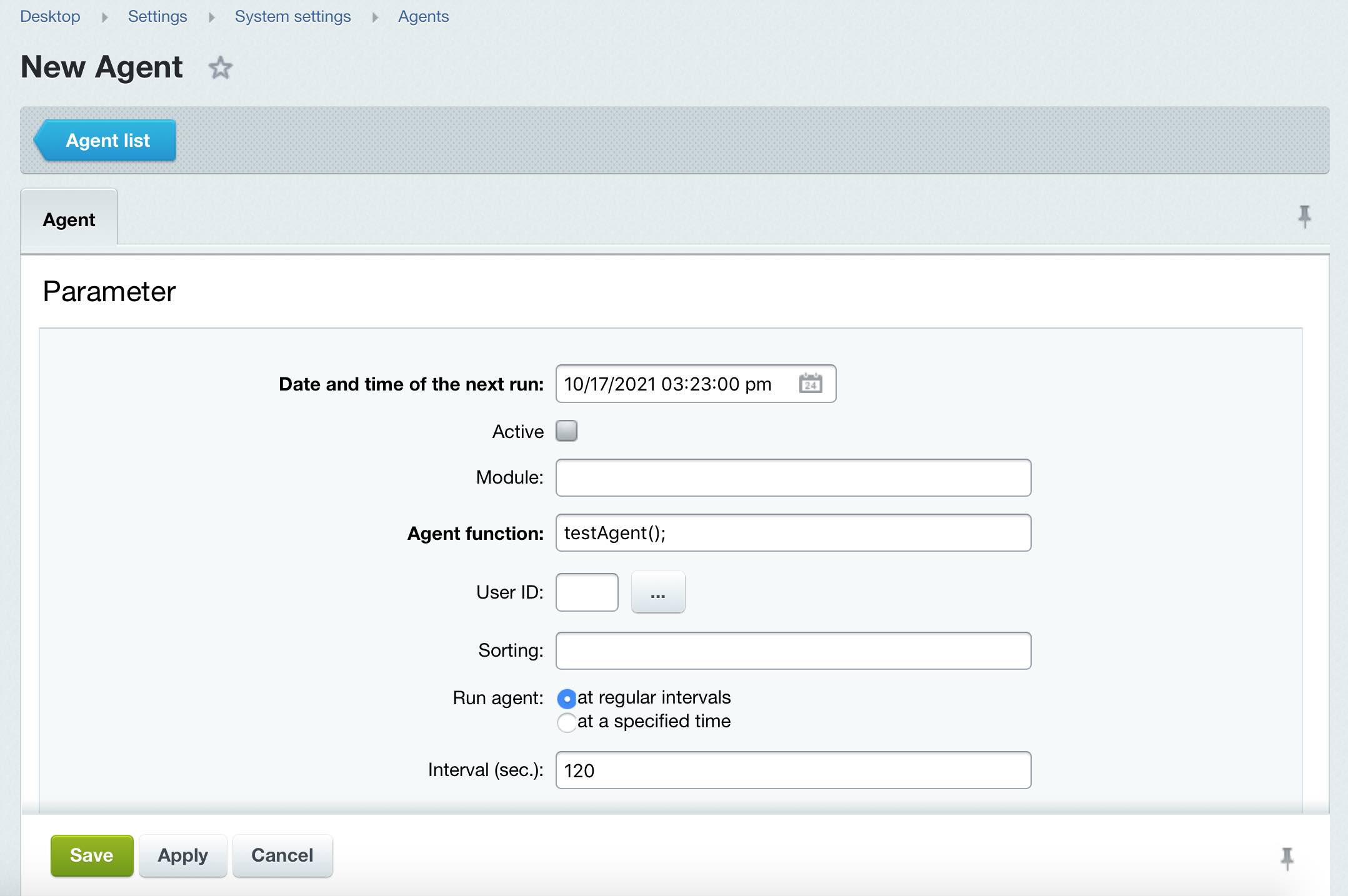
Some clarifications regarding parameters can be found below:
- Date of the last run - shows time of the most recent launch (when editing the agent);
- Date and time of the next run – time for agent start (in case of non-periodic agent); agent is executed once at this time;
- module - this module will be automatically connected, specifically connecting the file
/bitrix/modules/module_ID/include.php
. In this case, ensure that the agent function will be available after connecting this file;
- Agent function - this is the main field, with the function named testAgent();
- User ID – this is the filter for execution on the hit for specific user фильтр выполнения на хите для определенного пользователя;
The function itself will look as follows:
function testAgent()
{
mail('mail@gmail.com', 'Agent', 'Agent');
return "testAgent();";
}
You can dd the function to the file /bitrix/php_interface/init.php
.
To activate the agent, execute the following code in the admin section PHP console:
CAgent::AddAgent("testAgent();");
In case of a new email, the agent is triggered and you can add you own function.
Simple agent examples
<?
// add agent for the "Statistics" module
CAgent::AddAgent(
"CStatistic::CleanUpStatistics_2();", // function name
"statistic", // module identifier
"N", // agent is non-critical for the number launches
86400, // launch interval - 1 per day
"07.04.2005 20:03:26", // date of launch first check
"Y", // agent is active
"07.04.2005 20:03:26", // date of first launch
30);
?>
<?
// add agent for the "Helpdesk" module
CAgent::AddAgent(
"CTicket::AutoClose();", // function name
"support", // module identifier
"N", // agent is non-critical for the number launches
86400, // launch interval - 1 per day
"", // date of launch first check - current
"Y", // agent is active
"", // date of first launch - current
30);
?>
<?
// add a random agent not belonging to any module
CAgent::AddAgent("My_Agent_Function();");
?>
<?
// file /bitrix/php_interface/init.php
function My_Agent_Function()
{
// execute some actions
return "My_Agent_Function();";
}
?>
<?
// add a random agent, belonging to the module
// with identifier my_module
CAgent::AddAgent(
"CMyModule::Agent007(1)",
"my_module",
"Y",
86400);
?>
<?
// this agent will be launched precisely 7 times with the period once per day,
// after it will be deleted from the agent table.
Class CMyModule
{
public static function Agent007($cnt=1) : string
{
echo "Hello!";
if($cnt>=7)
return "";
return "CMyModule::Agent007(".($cnt+1).");";
}
}>
Currency rates
Practical example: Update a currency rate at the site. It's recommended to assign an agent to cron.
<?// Currency rate update
function AgentGetCurrencyRate()
{
global $DB;
// connect the 'currency' module
if(!CModule::IncludeModule('currency'))
return "AgentGetCurrencyRate();";
$arCurList = array('USD', 'EUR');
$bWarning = False;
$rateDay = GetTime(time(), "SHORT", LANGUAGE_ID);
$QUERY_STR = "date_req=".$DB->FormatDate($rateDay, CLang::GetDateFormat("SHORT", SITE_ID), "D.M.Y");
$strQueryText = QueryGetData("www.cbr.ru", 80, "/scripts/XML_daily.asp", $QUERY_STR, $errno, $errstr);
// this string is needed only for site having the encoding utf-8
$strQueryText = iconv('windows-1251', 'utf-8', $strQueryText);
if (strlen($strQueryText) <= 0)
$bWarning = True;
if (!$bWarning)
{
require_once($_SERVER["DOCUMENT_ROOT"]."/bitrix/modules/main/classes/general/xml.php");
$objXML = new CDataXML();
$objXML->LoadString($strQueryText);
$arData = $objXML->GetArray();
$arFields = array();
$arCurRate["CURRENCY_CBRF"] = array();
if (is_array($arData) && count($arData["ValCurs"]["#"]["Valute"])>0)
{
for ($j1 = 0; $j1<count($arData["ValCurs"]["#"]["Valute"]); $j1++)
{
$arFields = array(
"CURRENCY" => $arData["ValCurs"]["#"]["Valute"][$j1]["#"]["CharCode"][0]["#"],
'DATE_RATE' => $rateDay,
'RATE' => DoubleVal(str_replace(",", ".", $arData["ValCurs"]["#"]["Valute"][$j1]["#"]["Value"][0]["#"])),
'RATE_CNT' => IntVal($arData["ValCurs"]["#"]["Valute"][$j1]["#"]["Nominal"][0]["#"]),
);
CCurrencyRates::Add($arFields);
}
}
}
return "AgentGetCurrencyRate();";
}?>
Specified code is added to the file /bitrix/php_interface/init.php
. Do not forget to add the agent AgentGetCurrencyRate(); at the page Settings > System Settings > Agents.
Items without prices
Agent that checks the availability of iblock items without completed prices.
Let's develop a function that checks iblock items for completed prices. If such items are available, you need to send an email to the administrator regarding its amount and to add a corresponding entry into event log.
- Create:
- Email event and template.
- 'Agent', that will launch the function once per day.
- Make sure that agent is launched, log entry is created and email are received in the mailbox.
- Function must be located in a separate file that is connected in
init.php
.
function AgentChekPrice()
{
if(CModule::IncludeModule("iblock"))
{
$arSelect = Array("ID", "NAME", "PROPERTY_PRICE");
$arFilter = Array("IBLOCK_ID"=> 2, "PROPERTY_PRICE" => false);
$rsResCat = CIBlockElement::GetList(Array(), $arFilter, false, false, $arSelect);
$arItems = array();
while($arItemCat = $rsResCat->GetNext())
{
$arItems[] = $arItemCat;
}
CEventLog::Add(array(
"SEVERITY" => "SECURITY",
"AUDIT_TYPE_ID" => "CHECK_PRICE",
"MODULE_ID" => "iblock",
"ITEM_ID" => "",
"DESCRIPTION" => "Price check, no prices for ".count($arItems)." items",
));
if(count($arItems) > 0)
{
$arFilter = Array(
"GROUPS_ID" => Array(2)
);
$rsUsers = CUser::GetList(($by="personal_country"), ($order="desc"), $arFilter);
$arEmail = array();
while($arResUser = $rsUsers->GetNext())
{
$arEmail[] = $arResUser["EMAIL"];
}
if(count($arEmail) > 0)
{
$arEventFields = array(
"TEXT" => "Price check, no prices for ".count($arItems)." items",
"EMAIL" => implode(", ", $arEmail),
);
CEvent::Send("INFO_PRICE", "s1", $arEventFields);
}
}
}
return "AgentChekPrice();";
}